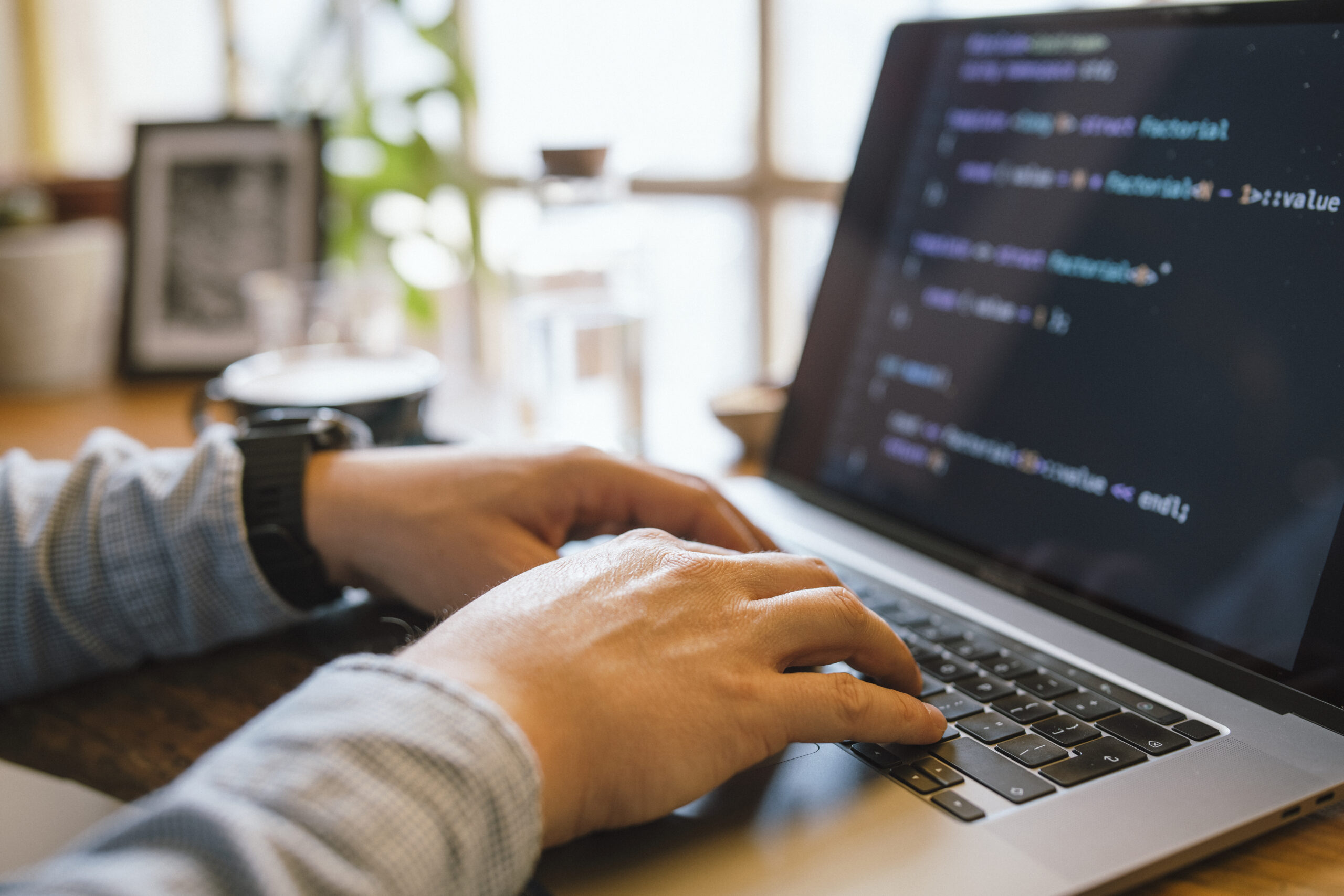
Debugging is One of the more crucial — nonetheless often ignored — expertise in the developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about understanding how and why issues go Improper, and Understanding to Consider methodically to resolve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of frustration and significantly improve your efficiency. Here are quite a few procedures that will help builders degree up their debugging game by me, Gustavo Woltmann.
Learn Your Applications
Among the list of fastest techniques developers can elevate their debugging expertise is by mastering the resources they use each day. While crafting code is one particular Portion of progress, being aware of how you can interact with it successfully for the duration of execution is equally significant. Present day advancement environments arrive equipped with effective debugging capabilities — but a lot of developers only scratch the surface area of what these resources can do.
Consider, as an example, an Built-in Progress Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools assist you to set breakpoints, inspect the worth of variables at runtime, action by means of code line by line, and even modify code about the fly. When employed correctly, they Enable you to observe exactly how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, see real-time functionality metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable responsibilities.
For backend or system-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Manage in excess of functioning processes and memory management. Finding out these applications may have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be comfortable with Edition Regulate systems like Git to know code historical past, uncover the precise instant bugs were being introduced, and isolate problematic alterations.
In the long run, mastering your instruments means going beyond default settings and shortcuts — it’s about producing an personal expertise in your enhancement environment so that when problems arise, you’re not missing in the dead of night. The greater you already know your instruments, the greater time it is possible to commit resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Among the most important — and sometimes neglected — methods in powerful debugging is reproducing the challenge. Ahead of leaping in the code or producing guesses, developers need to produce a regular setting or circumstance in which the bug reliably appears. Without the need of reproducibility, repairing a bug gets a sport of chance, usually leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is accumulating as much context as you can. Request questions like: What steps resulted in The difficulty? Which natural environment was it in — progress, staging, or output? Are there any logs, screenshots, or mistake messages? The more element you might have, the simpler it results in being to isolate the exact conditions under which the bug happens.
When you’ve gathered sufficient information and facts, try and recreate the problem in your neighborhood setting. This may suggest inputting the same knowledge, simulating similar consumer interactions, or mimicking method states. If The problem seems intermittently, take into account writing automated assessments that replicate the sting scenarios or state transitions concerned. These checks not only support expose the condition but additionally protect against regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on specified functioning systems, browsers, or below distinct configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser tests platforms could be instrumental in replicating these types of bugs.
Reproducing the trouble isn’t merely a move — it’s a mindset. It demands tolerance, observation, and a methodical method. But after you can continually recreate the bug, you happen to be now midway to correcting it. Having a reproducible situation, You need to use your debugging equipment far more proficiently, take a look at probable fixes safely, and communicate more clearly with your team or users. It turns an summary complaint into a concrete challenge — Which’s exactly where developers prosper.
Examine and Fully grasp the Mistake Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, developers ought to learn to take care of mistake messages as direct communications in the system. They normally inform you what precisely took place, in which it happened, and in some cases even why it took place — if you understand how to interpret them.
Start by examining the information meticulously and in full. Quite a few developers, especially when underneath time stress, glance at the first line and straight away start out producing assumptions. But further while in the error stack or logs may well lie the legitimate root lead to. Don’t just duplicate and paste error messages into search engines like yahoo — browse and recognize them initial.
Split the error down into parts. Could it be a syntax mistake, a runtime exception, or possibly a logic mistake? Does it issue to a particular file and line quantity? What module or purpose induced it? These thoughts can information your investigation and point you toward the liable code.
It’s also useful to know the terminology with the programming language or framework you’re utilizing. Error messages in languages like Python, JavaScript, or Java normally stick to predictable designs, and learning to recognize these can considerably accelerate your debugging system.
Some mistakes are obscure or generic, As well as in those circumstances, it’s very important to examine the context where the mistake occurred. Examine linked log entries, enter values, and recent adjustments from the codebase.
Don’t neglect compiler or linter warnings both. These generally precede bigger concerns and supply hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint troubles speedier, cut down debugging time, and turn into a more efficient and confident developer.
Use Logging Correctly
Logging is One of the more powerful tools in a developer’s debugging toolkit. When utilized successfully, it provides genuine-time insights into how an software behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
A superb logging approach starts off with figuring out what to log and at what amount. Popular logging degrees include things like DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for in depth diagnostic details throughout improvement, INFO for typical gatherings (like prosperous start off-ups), WARN for likely concerns that don’t break the applying, Mistake for real problems, and Lethal if the method can’t proceed.
Steer clear of flooding your logs with excessive or irrelevant facts. Excessive logging can obscure crucial messages and decelerate your technique. Give attention to key occasions, point out improvements, input/output values, and important determination points as part of your code.
Format your log messages Evidently and constantly. Incorporate context, such as timestamps, ask for IDs, and performance names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Throughout debugging, logs Permit you to track how variables evolve, what ailments are satisfied, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. That has a properly-thought-out logging technique, you could reduce the time it will require to identify problems, achieve further visibility into your applications, and Increase the overall maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not only a complex undertaking—it is a sort of investigation. To correctly determine and resolve bugs, builders ought to solution the process like a detective fixing a thriller. This mindset aids break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by accumulating proof. Look at the symptoms of the issue: error messages, incorrect output, or efficiency troubles. Similar to a detective surveys a criminal offense scene, accumulate just as much applicable information as you can with out jumping to conclusions. Use logs, exam cases, and person experiences to piece alongside one another a transparent photograph of what’s going on.
Upcoming, sort hypotheses. Check with by yourself: What may be leading to this conduct? Have any modifications recently been created towards the codebase? Has this problem happened in advance of underneath equivalent situations? The goal should be to slim down prospects and establish likely culprits.
Then, check your theories systematically. Try to recreate the condition in a very controlled environment. When you suspect a particular function or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Fork out shut focus to small facts. Bugs usually disguise inside the the very least predicted places—just like a missing semicolon, an off-by-one error, or a race issue. Be thorough and individual, resisting the urge to patch the issue with no fully comprehension it. Temporary fixes may possibly cover the real dilemma, only for it to resurface later on.
Last of all, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can save time for foreseeable future challenges and aid Some others understand your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic complications methodically, and turn out to be more practical at uncovering hidden problems in intricate units.
Write Exams
Producing checks is among the most effective strategies to enhance your debugging expertise and Over-all development efficiency. Tests not just support capture bugs early and also function a security Web that provides you self confidence when building variations to your codebase. A well-tested application is easier to debug because it allows you to pinpoint precisely exactly where and when a challenge takes place.
Begin with unit exams, which give attention to specific features or modules. These tiny, isolated exams can rapidly reveal whether or not a certain piece of logic is Operating as expected. When a exam fails, you straight away know where by to glance, appreciably minimizing time invested debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, integrate integration tests and close-to-conclusion tests into your workflow. These enable be certain that different elements of your software get the job done collectively smoothly. They’re significantly valuable for catching bugs that happen in elaborate systems with many elements or services interacting. If a thing breaks, your exams can show you which Section of the pipeline unsuccessful and beneath what circumstances.
Producing exams also forces you to definitely Consider critically about your code. To check a function adequately, you will need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of being familiar with In a natural way leads to higher code composition and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails continuously, you'll be able to deal with fixing the bug and observe your check move when The difficulty is resolved. This technique makes certain that the same bug doesn’t return Later on.
Briefly, writing tests turns debugging from a discouraging guessing activity into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Consider Breaks
When debugging a tricky situation, it’s uncomplicated to be immersed in the problem—staring at your display for hrs, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your intellect, cut down irritation, and infrequently see The difficulty from the new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious faults or misreading code that you wrote just several hours before. During this point out, your brain results in being fewer economical at challenge-fixing. A short walk, a espresso crack, as well as switching to a distinct job for 10–quarter-hour can refresh your concentration. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function in the history.
Breaks also support avoid burnout, especially all through extended debugging classes. Sitting in front of a display screen, mentally stuck, is don't just unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer mindset. You would possibly out of the blue discover a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded read more you prior to.
For those who’re caught, a good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that point to move around, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, especially beneath limited deadlines, nevertheless it basically contributes to a lot quicker and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a smart tactic. It gives your brain Place to breathe, increases your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is an element of resolving it.
Discover From Each Bug
Each and every bug you face is more than just A brief setback—It truly is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or perhaps a deep architectural concern, each can train you a little something valuable should you go to the trouble to reflect and evaluate what went Improper.
Start off by inquiring on your own a handful of key concerns once the bug is resolved: What brought on it? Why did it go unnoticed? Could it have already been caught previously with greater techniques like device tests, code reviews, or logging? The answers often reveal blind places in the workflow or being familiar with and help you build stronger coding habits going ahead.
Documenting bugs can even be an outstanding practice. Retain a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll begin to see styles—recurring troubles or frequent blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your friends might be Specifically powerful. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a much better Finding out tradition.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. Rather than dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In any case, a lot of the ideal builders will not be those who compose fantastic code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you correct provides a fresh layer towards your talent established. So up coming time you squash a bug, have a moment to mirror—you’ll appear absent a smarter, a lot more able developer because of it.
Conclusion
Strengthening your debugging skills will take time, exercise, and patience — nevertheless the payoff is large. It makes you a more productive, self-assured, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at Everything you do.